#Fixed Deposits SDK integration guide for Android
#Add a button
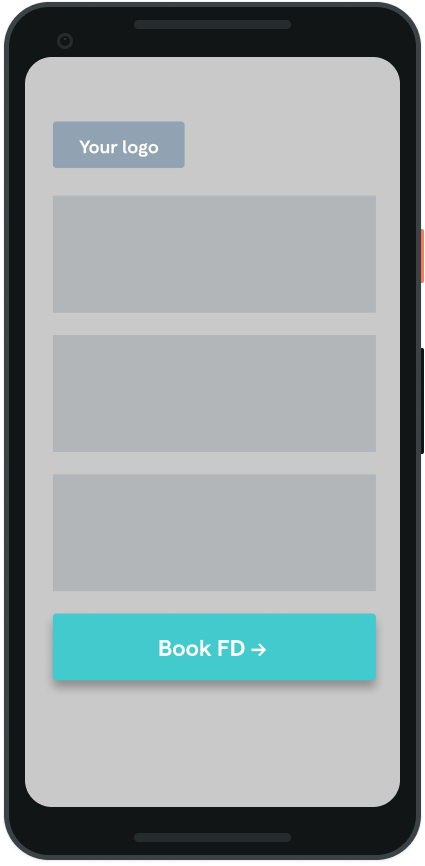
Add the following button at an appropriate location to your activity.
#Important notes
- A valid SDK
secretKey
obtained from the bridge under-investment product has to be passed assecretKey
in the function call. - Provide
env
asMOCK
for mock environment andPROD
for production environment. - Provide these values to theme some parts of the SDK—
logoUrl
link to your logo
- Provide
redirectTo
as the URL object to which the user should be redirected after the transaction is completed.- on success or failure, we will redirect user to
success
orfailure
URL, provided inredirectTo
object with a query parameterapplicationId
with value as the application ID of the transaction. - this will help in easier way to map transactions with respect to one user and to check when one user has created multiple FDs with different providers
- on success or failure, we will redirect user to
provider
is the bank/NBFC offering the FD. Following are the values for various bank/NBFC partners—
provider | provider code |
---|---|
Ujjivan Small Finance Bank | UJVN |
Bajaj Finance NBFC | BAJAJ |
All prefills aren't mandatory. Only email
, amount
, env
and tenure
are mandatory. If you choose to skip a prefill, simply don't include it in your code.
Note that the key provider
has a value of UJVN
, representing the FD providing bank.
Provide tenure
in days. For example, if you want to provide a tenure of 180 days, provide tenure: 180
. The range of tenure is 180 to 365 days.
Note that the key env
has a value of MOCK
, representing the current environment. The possible values are MOCK
, UAT
and PROD
.
To re-initate the SDK for user to restart from where the user left. You need to call this function once again.
This is a sample implementation of invoking our FD SDK in Android.
Android implementation using Java
package com.example.deposits; // Replace with your package nameimport androidx.appcompat.app.AppCompatActivity;import android.annotation.SuppressLint;import android.os.Bundle;import android.util.Base64;import android.webkit.WebView;import android.webkit.WebViewClient;import org.json.JSONException;import org.json.JSONObject;public class MainActivity extends AppCompatActivity {@Overrideprotected void onCreate(Bundle savedInstanceState) {super.onCreate(savedInstanceState);setContentView(R.layout.activity_main);WebView webView = getWebviewObject();// HTML to load SDKString html = "<script type="text/javascript" src="https://fd.setu.co/sdk.js"></script><p>Loading...</p>";String encodedHtml = Base64.encodeToString(html.getBytes(),Base64.NO_PADDING);// Set prefills hereJSONObject prefills = new JSONObject();try {prefills.put("secretKey", "YOUR_SECRET_KEY");prefills.put("env", "MOCK"); // MOCK, PRODprefills.put("partnerName", "YOUR_PARTNER_NAME");prefills.put("theme", getPrefillsTheme());prefills.put("provider", "UJVN");prefills.put("prefills", getPrefills());prefills.put("personalParams", getPersonalParams());prefills.put("nomineeParams", getNomineeParams());prefills.put("maturityParams", getMaturityParams());prefills.put("redirectTo", getRedirectionDetails());} catch (JSONException e) {e.printStackTrace();}String jsString = "(function handleClick(){document.Setu.FD.init(%s)})();";String injectableJS = String.format(jsString, prefills);webView.loadData(encodedHtml, "text/html", "base64");webView.setWebViewClient(new WebViewClient() {@Overridepublic void onPageFinished(WebView view, String url) {if (view.getUrl().startsWith("data:")){view.evaluateJavascript( injectableJS, paRes -> {// Handle response here});});}super.onPageFinished(view, url);}});}// Set themeJSONObject getPrefillsTheme() throws JSONException {JSONObject theme = new JSONObject();theme.put("logoUrl", "https://image.shutterstock.com/image-vector/abstract-vector-logo-design-template-600w-1971786323.jpg");theme.put("primaryColor", "#3742FA");theme.put("secondaryColor", "#E2ECFC");theme.put("primaryTextColor", "#1A202C");theme.put("secondaryTextColor", "#A0AEC0");theme.put("hoverOnPrimaryColor", "#2C3AFA");theme.put("inputFieldBackgroundColor", "#F7FAFC");theme.put("inputFieldBorderColor", "#3742FA");theme.put("cardColor", "#FFFFFF");theme.put("globalBackgroundColor", "#F7FAFC");return theme;}// Set amount, tenue and KYC paramsJSONObject getPrefills() throws JSONException {JSONObject prefils = new JSONObject();prefils.put("amount", 25000);prefils.put("tenure", 180);JSONObject kyc = new JSONObject();kyc.put("email", "ramesh@xyz.com");kyc.put("pan", "ABCD1234E");kyc.put("mobile", "9998887776");prefils.put("kycParams", kyc);return prefils;}// Set personal paramsJSONObject getPersonalParams() throws JSONException {JSONObject personal = new JSONObject();personal.put("mothersName", "Rhea");personal.put("fathersName", "Kronos");personal.put("maritalStatus", "MARRIED");personal.put("spouseName", "Hera");personal.put("qualification", "10TH");personal.put("occupation", "EMPLOYED");personal.put("designation", "SALARIED");personal.put("communicationAddress", "6th main, Subhash Nagar, Tumkur");personal.put("communicationPinCode", "560023");return personal;}// Set nominee paramsJSONObject getNomineeParams() throws JSONException {JSONObject nomineeParams = new JSONObject();nomineeParams.put("nomineeRelationship", "SON");nomineeParams.put("nomineeName", "Suresh");nomineeParams.put("nomineeDob", "1992-11-11");nomineeParams.put("nomineeEmail", "suresh@xyz.com");nomineeParams.put("nomineePincode", "560023");return nomineeParams;}// Set account detailsJSONObject getMaturityParams() throws JSONException {JSONObject maturityParams = new JSONObject();maturityParams.put("maturityIfsc", "SBIN0050432");maturityParams.put("maturityAccountNumber", "00112233445566");return maturityParams;}// Set redirection objectJSONObject getRedirectionDetails() throws JSONException {JSONObject redirectTo = new JSONObject();redirectTo.put("success", "https://my-website.co/success");redirectTo.put("failure", "https://my-website.co/failure");return redirectTo;}@SuppressLint("SetJavaScriptEnabled")WebView getWebviewObject(){WebView webView = findViewById(R.id.webview);webView.getSettings().setJavaScriptEnabled(true);webView.getSettings().setJavaScriptCanOpenWindowsAutomatically(true);webView.getSettings().setDomStorageEnabled(true);return webView;}}
#Mock values for testing
Once you have successfully embedded the SDK on your page, use the following input values for testing the SDK journey in sandbox—

Verify your details screen
Use 111111 as the OTP for verifying mobile number and ABCD1234E as the PAN for verifying PAN.
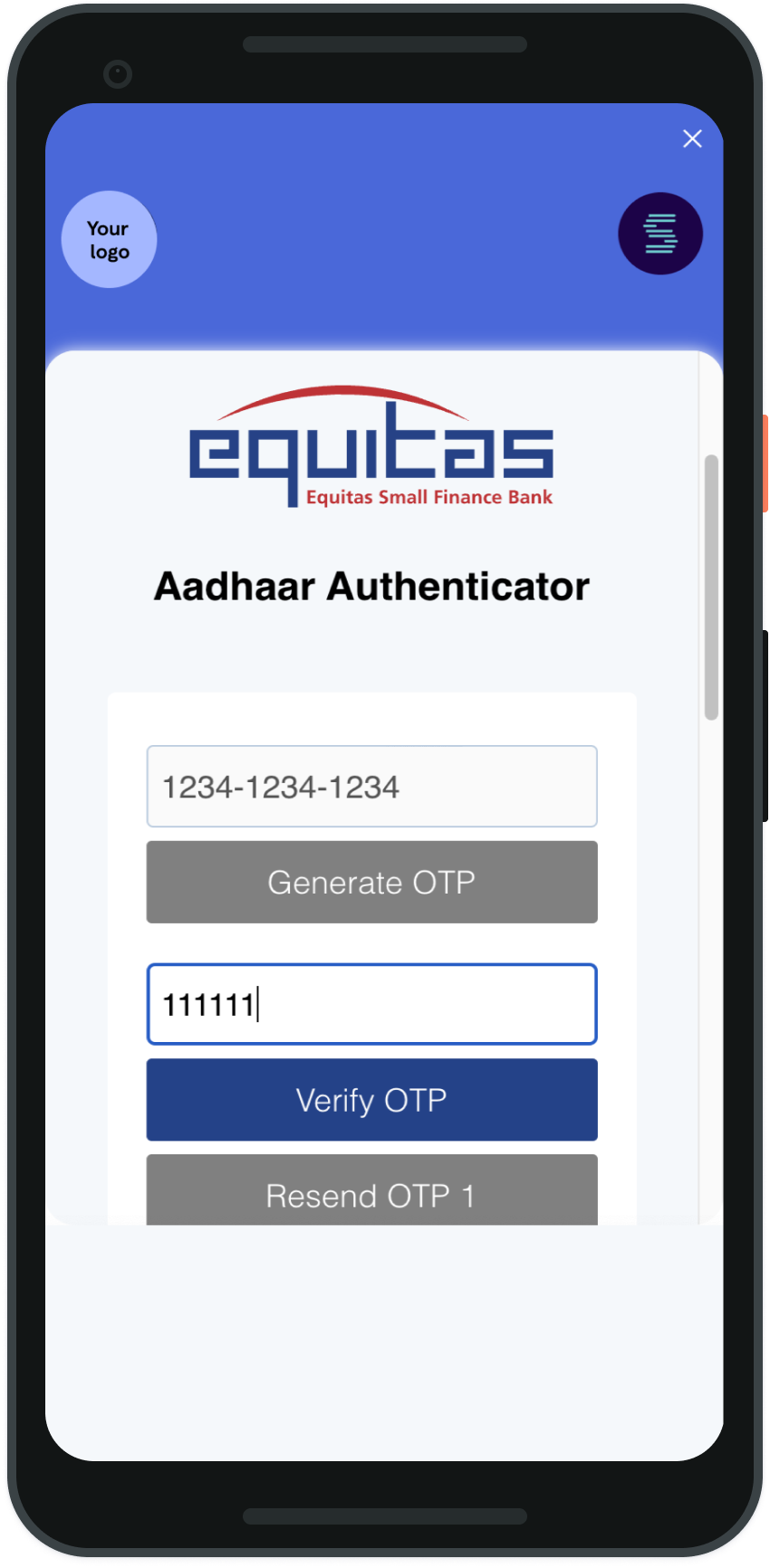
Verify your Aadhaar screen
Use 1234-1234-1234 as the Aadhaar number and 111111 as the OTP for verifying mobile number.

Mock payment screen
On the payment page, please wait for a while—the payment is automatically mocked.
Was this page helpful?